Advanced C# |
Course Outline |
Outline
|
Reading Material |
Reference.pdf
|
PowerPoint Slides |
Intro.pptx
|
Day | Modules | Sharing |
1 |
- Information Hiding
- OOP
- More advance C# features
|
|
2 |
- Overview of Attributes
- Defining Custom Attributes
- Retrieving Attribute Values
|
|
- Reflecting on Objects
- Adding Assembly Metadata
- Emitting Objects by Using Builder Classes
|
|
- Comparing a Static Method with an Instance Method
- Single Cast Delegates
- Multicast Delegates
- Variance in Delegates
|
|
3 |
- Creating Custom Events
- Passing Data to an Event Argument
- Using Event Accessors
- Handling Interface Events
- Handling Explicit Interface Events
|
|
- The Usefulness of Lambda Expressions
- Lambda Expression with (and Without) Parameters
- Types of Lambda Expressions
- Expression-Bodied Members
- Local Variables in a Lambda Expression
- Using Tuples in a Lambda Expression
- Event Subscription with Lambda Expressions
|
|
- The Motivation Behind Generics
- A Quick Look into the List Class
- Generic Delegates
- Predicate Delegate
- The Default Keyword in Generics
- Implementing Generic Interface
- Generic Constraints
- Using Covariance and Contravariance
- Covariance with Generic Delegate
- Covariance with Generic Interfaces
- Contravariance with Generic Delegates
- Contravariance with Generic Interface
- Self-Referencing Generic Types
|
|
4 |
- Foundations in Thread Programming
- Coding Multithreaded Programs in C#
- Using the ThreadStart Delegate
- Using the ParameterizedThreadStart Delegate
- Foreground Thread vs. Background Thread
- Thread Safety
- An Alternative Approach Using the Monitor Class
- Deadlock
|
|
- Using a Synchronous Approach
- Using Thread Class
- Using the ThreadPool Class
- Using Lambda Expressions with ThreadPool
- Using the IAsyncResult Pattern
- Using an Event-based Asynchronous Pattern (EAP)
- Understanding Tasks
- Using a Task-based Asynchronous Pattern (TAP)
- Asynchronous Programming with Async / Await
|
|
- C# Features That Support LINQ
- Query Syntax and Method Syntax in LINQ
- Basic LINQ Query Operations
- Data Transformations with LINQ
|
|
Recommanded Books |
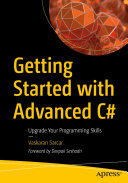 | Title | Getting Started with Advanced C#: Upgrade Your Programming Skills |
ISBN | 978-1-48-425934-4 |
Author | Vaskaran Sarcar |
Year | 2020 |
Publisher | Apress |
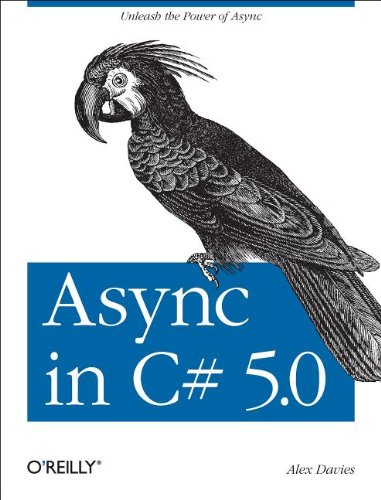 | Title | Async in C# 5.0 |
ISBN | 978-1-449-33716-2 |
Author | Alex Davies |
Year | 2012 |
Publisher | O'Reilly Media |