Refactoring to Patterns using C# |
Course Outline |
Outline
|
Reading Material |
|
PowerPoint Slides |
Intro.pptx
|
Day | Modules | Sharing |
1 |
- The Patterns Panacea
- Under-Engineering
- Test-Driven Development and Continuous Refactoring
- Refactoring and Patterns
- Evolutionary Design
|
|
- What Is Refactoring?
- The motivations to refactor
- Human-Readable Code
- Keeping It Clean
- Small Steps
- Design Debt
- Evolving a New Architecture
- Composite and Test-Driven Refactorings
- The Benefits of Composite Refactorings
- Refactoring Tools
|
|
- What Is a Pattern?
- Very brief introduction to the GoF Patterns
- There Are Many Ways to Implement a Pattern
- Refactoring to, towards, and away from Patterns
- Do Patterns Make Code More Complex?
- Pattern Knowledge
- Up-Front Design with Patterns
|
|
- Duplicated Code
- Long Method
- Conditional Complexity
- Primitive Obsession
- Indecent Exposure
- Solution Sprawl
- Alternative Classes with Different Interfaces
- Lazy Class
- Large Class
- Switch Statements
- Combinatorial Explosion
- Oddball Solution
|
|
2 |
- Format of the Refactorings
- Projects Referenced in This Catalog
- A Starting Point
- A Study Sequence
|
|
- Replace Constructors with Creation Methods
- Move Creation Knowledge to Factory
- Encapsulate Classes with Factory
- Introduce Polymorphic Creation with Factory Method
- Encapsulate Composite with Builder
- Inline Singleton
|
|
- Compose Method
- Replace Conditional Logic with Strategy
- Move Embellishment to Decorator
- Replace State-Altering Conditionals with State
- Replace Implicit Tree with Composite
- Replace Conditional Dispatcher with Command
|
|
- Form Template Method
- Extract Composite
- Replace One-Many Distinctions with Composite
- Replace Hard-Coded Notifications with Observer
- Unify Interfaces with Adapter
- Extract Adapter
- Replace Implicit Language with Interpreter
|
|
3 |
- Replace Type Code with Class
- Limit Instantiation with Singleton
- Introduce Null Object
|
|
- Move Accumulation to Collecting Parameter
- Move Accumulation to Visitor
|
|
- Chain Constructors
- Unify Interfaces
- Extract Parameter
|
|
Recommanded Books |
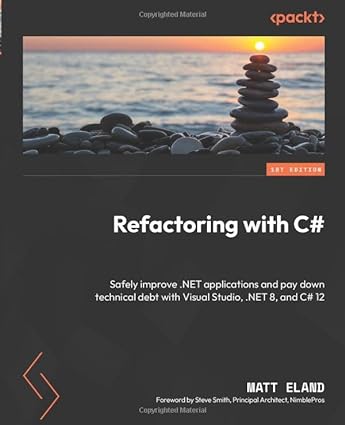 | Title | Refactoring with C#: Safely improve .NET applications and pay down technical debt with Visual Studio, .NET 8, and C# 12 |
ISBN | 978-1-835-08998-9 |
Author | Matt Eland |
Year | 2024 |
Publisher | Packt Publishing Pvt Ltd |
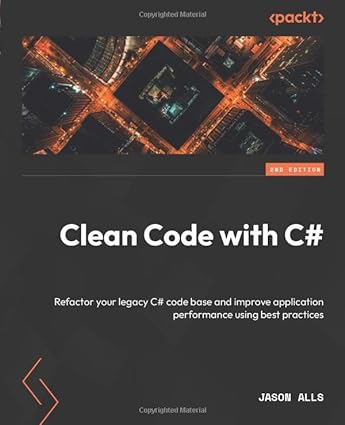 | Title | Clean Code with C#: Refactor your legacy C# code base and improve application performance using best practices |
ISBN | 978-1-837-63519-1 |
Author | Jason Alls |
Year | 2023 |
Publisher | Packt Publishing |